Implementation of CPU scheduling algorithms to find turnaround time and waiting time.
Burst Time : Every process in a computer system requires some amount of time for its execution. Burst time is the total time taken by the process for its execution on the CPU.
Waiting Time : Waiting time is the total time spent by the process in the ready state waiting for CPU.
Waiting Time = Turn Around Time – Burst Time
Arrival Time : Arrival time is the time when a process enters into the ready state and is ready for its execution.
Turnaround Time : Turnaround time is the total amount of time spent by the process from coming in the ready state for the first time to its completion.
Turnaround time = Burst time + Waiting time or Turnaround time = Exit time – Arrival time
Program
#include<iostream.h> #include<conio.h> #define MAXLIMIT 20 void main(){ clrscr(); int n,burst_time[20],waiting_time[20],turnaround_time[20],avg_waiting_time=0,avg_turnaround_time=0,i,j; cout<<"Enter Total Number of Processes (Max 20): "; cin>>n; //Number of Process if(n>=MAXLIMIT){ cout<<"----Sorry :( MaxLimit is 20 You Enter "<<n<<" ----"; //End If }else{ cout<<"\nEnter Process Burst Time\n"; for(i=0;i<n;i++) { cout<<"P["<<i+1<<"]: "; cin>>burst_time[i]; } waiting_time[0]=0; //Waiting Time for First Process is 0 //Calculating Waiting Time for(i=1;i<n;i++){ waiting_time[i]=0; for(j=0;j<i;j++) waiting_time[i]+=burst_time[j]; } cout<<"\nProcess\t\tBurst Time\tWaiting Time\tTurnaround Time"; //Calculating Turnaround time for(i=0;i<n;i++){ turnaround_time[i] = burst_time[i]+waiting_time[i]; avg_waiting_time += waiting_time[i]; avg_turnaround_time += turnaround_time[i]; cout<<"\nP["<<i+1<<"]"<<"\t\t"<<burst_time[i]<<"\t\t"<<waiting_time[i]<<"\t\t"<<turnaround_time[i]; } avg_waiting_time/=i; avg_turnaround_time/=i; cout<<"\n\nAverage Waiting Time:"<<avg_waiting_time; cout<<"\nAverage Turnaround Time:"<<avg_turnaround_time; }//End Else getch(); //Hold Program Execution For Display }
Program Output
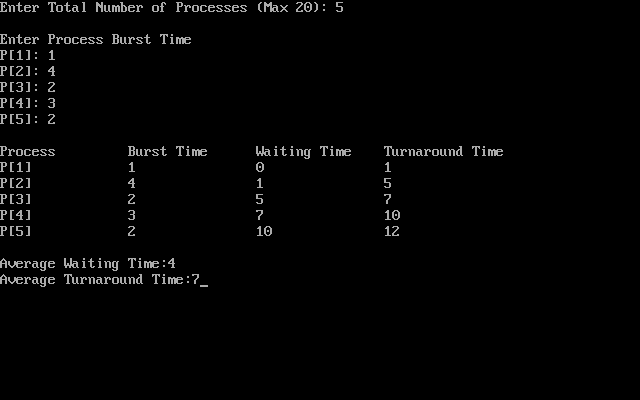
(Visited 275 times, 1 visits today)
Written by: