What is DataTypes ?
An data types is used to hold different types of data or values.In PHP supports 8 primitive data types.
Different DataTypes
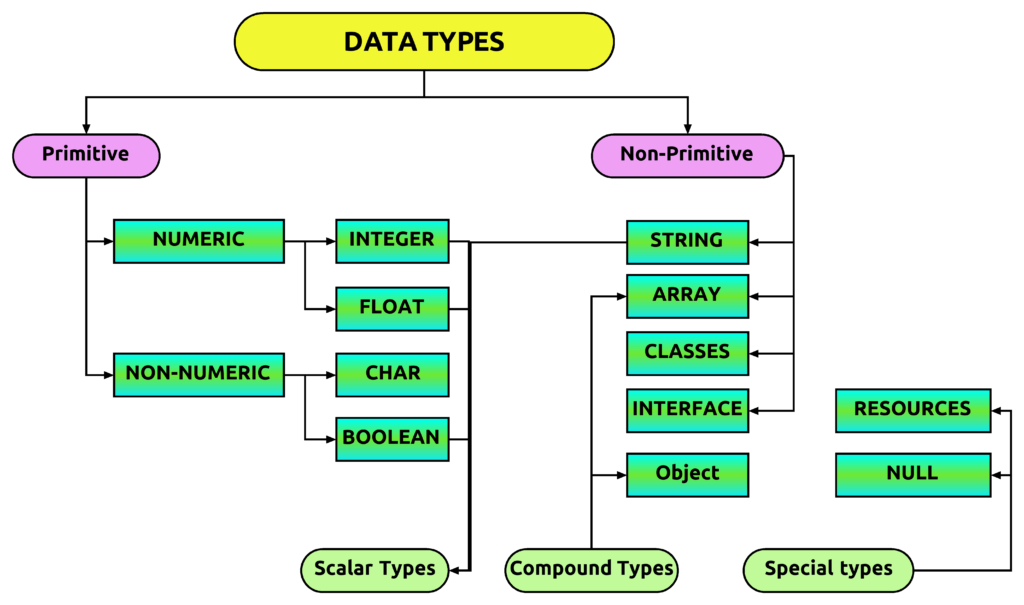
- Scalar Types (predefined)
- Boolean
- Integer
- Float
- String
- Compound Types (user-defined)
- Array
- Object
- Special Types
- Resource
- NULL
Boolean
A boolean expresses a truth value. Boolean: Hold only two values, either TRUE or FALSE.
Example
<?php $data1 = true; $data2 = 12; // Since $data1 is a boolean, it will return true if (is_bool($data1) === true) { echo "Data1 Yes, this is a boolean"; } // Since $data2 is not a boolean, it will return false output not show if (is_bool($data2) === true) { echo "Data2 Yes, this is a boolean"; } /* Output Data1 Yes, this is a boolean */ ?>
Integer
An integer is a decimal number, decimal (base 10), hexadecimal (base 16), octal (base 8) or binary (base 2) notation.
Example : 1,2,3,4,5 etc
<?php $data1 = 123456; $data2 = false; // $data1 is a integer, it will return true if (is_int($data1) === true) { echo "Data1 Yes, this is a integer"; } // $data2 is not a integer, it will return false output not show if (is_int($data2) === true) { echo "Data2 Yes, this is a integer"; } /* Output Data1 Yes, this is a integer */ ?>
Float
A Float is no fixed number of digits before and after the decimal point. The decimal point can float.
Example : 1.5, 2.5, 5.5, 0.001, and -5,345.6789 are floating point numbers.
<?php $data1 = 3.14; $data2 = 123; // $data1 is a float, it will return true if (is_float($data1) === true) { echo "Data1 Yes, this is a float"; } // $data2 is not a float, it will return false output not show if (is_float($data2) === true) { echo "Data2 Yes, this is a float"; } /* Output Data1 Yes, this is a float */ ?>
String
A String Hold letters or any alphabets, even numbers are included. These are written within double quotes during declaration. The strings can also be written within single quotes but it will be treated differently while printing variables.
Single Quotes ‘ ‘ inside $variable not work.
Double Quotes ” ” inside $variable work.
Example : “Hi “,’String ‘ etc
<?php $data1 = "String Text"; $data2 = 123; // $data1 is a String, it will return true if (is_string($data1) === true) { echo "Data1 Yes, this is a String"; } // $data2 is not a String, it will return false output not show if (is_string($data2) === true) { echo "Data2 Yes, this is a String"; } /* Output Data1 Yes, this is a String */ ?>
Arrays
Array is a compound data-type which can store multiple values of same data type. In PHP Array is actually an ordered map. A map is a type that associates values to keys. With keys call the values.
Example :
$Array = array(‘this’, ‘is’, ‘an array’);
<?php $Array_1 = array('this', 'is', 'an array'); $Array_2 = "String Text"; // $Array_1 is a array, it will return true if (is_array($Array_1) === true) { echo "Array1 Yes, this is a Array"; } // $Array_2 is not a array, it will return false output not show if (is_array($Array_2) === true) { echo "Array2 Yes, this is a Array"; } /* Output Array1 Yes, this is a Array */ ?>
Object
Objects are defined as instances of user defined classes that can hold both values and functions. This is an advanced topic and will be discussed in details in further articles.
Example : $new_obj = new programming; // object variable = new classname;
<?php class programming{ function learning(){ echo "Start Learning..."; } } $obj = 1; $new_obj = new programming; $new_obj->learning(); // $new_obj is a Object, it will return true if (is_object($new_obj) === true) { echo "New_obj Yes, this is a Object"; } // $obj is not a Object, it will return false output not show if (is_object($obj) === true) { echo "Obj Yes, this is a Object"; } /* Output New_obj Yes, this is a Object */ ?>
Resource
Resources in PHP are not an exact data type. These are basically used to store references to some function call or to external PHP resources. Example an active resource is a resource.
Example
$handle = fopen('text.txt', 'r'); echo (is_resource($handle))? "File Reserved Resources ": "File Reserved Resources Free"; // File Reserved Resources fclose($handle); echo (is_resource($handle))? "File Reserved Resources ": "File Reserved Resources Free"; //File Reserved Resources Free
NULL
The NULL is special types of variables that can hold only one value Example, NULL. the Null writing it in capital form, but its case sensitive.NULL means empty value.
Example
$value1 = Null; $value2 = 1; // $value1 is a Null, it will return true if (is_null($value1) === true) { echo "Value1 Yes, this is a Null"; } // $value2 is a not a NULL, it will return False // Output not display if (is_null($value2) === true) { echo "Value2 Yes, this is a Null"; } /* Output Value1 Yes, this is a Null */
Data Types in Table Form
Name | Type | Properties | Function To Check | Description |
Boolean | Scalar Types (predefined) | TRUE or FALSE true or false | is_bool() | Finds out whether a variable is a boolean |
Integer | Scalar Types (predefined) | 1 2 3 | is_int() | Find whether the type of a variable is integer |
Float | Scalar Types (predefined) | 0.1 2.32 | is_float() | Finds whether the type of a variable is float |
String | Scalar Types (predefined) | “String” or ‘abc’ | is_string() | Find whether the type of a variable is string |
Array | Compound Types (user-defined) | $yes = array(‘this’, ‘is’, ‘an array’); | is_array() | Finds whether a variable is an array |
Object | Compound Types (user-defined) | new stdClass | is_object() | Finds whether a variable is an object |
Resource | Special Types | is_resource() | Finds whether a variable is a resource | |
NULL | Special Types | NULL | is_null() | Finds whether a variable is NULL |